Efficient Array Emptiness Checks in JavaScript 5 Best Practices for 2024
Efficient Array Emptiness Checks in JavaScript 5 Best Practices for 2024 - Array isArray and length property combination
When it comes to efficiently determining if a JavaScript array is empty, a powerful approach is to couple the `Array.isArray` method with the `length` property. `Array.isArray` serves as a crucial first step, ensuring the variable you're inspecting is indeed an array. This guards against potential issues caused by unexpected data types. Once confirmed as an array, checking if its `length` property is equal to 0 provides a straightforward and reliable way to identify emptiness.
By consolidating these two checks into a dedicated function, such as `isEmpty(array)`, you promote cleaner and more reusable code. This compact function encapsulates the core logic, improving code readability and making it easier to maintain. While other techniques for array emptiness checks exist, they tend to introduce unnecessary complexity for a simple task like this. Utilizing this straightforward combination of `Array.isArray` and `length` remains the most efficient and pragmatic solution, especially when aiming for a clean and dependable way to evaluate array emptiness.
1. While `typeof` might seem sufficient for a quick check, `Array.isArray()` provides a more precise type validation, preventing potential issues where `typeof` might mistakenly classify arrays as objects. This heightened accuracy is invaluable for maintaining code integrity, particularly in debugging and troubleshooting situations.
2. The `length` property, while typically representing the number of elements, isn't inherently tied to the actual number of elements in an array. It's a dynamic property that can be manually altered, even set to a value smaller than the current number of elements, effectively truncating the array in an unusual manner. This can lead to unexpected outcomes if not carefully managed.
3. It's interesting to note that arrays, fundamentally, are specialized objects in JavaScript. This means their `length` property can be modified independently of the elements themselves. For instance, setting `length` to a smaller value than the actual element count essentially cuts off the array without removing those elements, a feature that's useful in specific scenarios but can be perplexing if not understood.
4. When combining `Array.isArray()` and `length` to ascertain array emptiness, as in `Array.isArray(arr) && arr.length === 0`, you're gaining a strong foundation in type safety while also maximizing efficiency. The length check itself is an O(1) operation, contributing to quick and consistent emptiness checks.
5. The existence of `Array.isArray()` underscores the crucial role of the internal `[[Class]]` property in JavaScript. It's the engine behind JavaScript's ability to differentiate arrays from other objects. This underlying mechanism is crucial for type enforcement and helps establish a clear boundary for what constitutes an array.
6. Although developers often might feel the urge to double-check the `length` property after checking for an array type, such redundant checks can introduce performance overheads, especially if occurring within tight loops. It's a trade-off where careful optimization comes into play, especially in performance-sensitive scenarios.
7. When used on non-iterable objects, `Array.isArray()` reliably returns `false`, making it a crucial component in functions designed to manipulate arrays. It serves as a security check, preventing unintended behavior and ensuring that only valid array inputs are accepted.
8. Pairing `Array.isArray()` with a `length` check doesn't just lead to a functional check for emptiness; it also significantly enhances readability. The code conveys intent more precisely and directly than complex logic that relies on multiple type checks and conditions.
9. Beyond merely identifying empty arrays, understanding the way `length` operates helps us construct custom data structures that resemble arrays in function. By leveraging this property, we can engineer optimized data structures to meet the unique needs of specific applications.
10. While setting an array's `length` property to zero seems like a simple way to empty it, it's worth noting that it might also spark a garbage collection process if those elements aren't referenced elsewhere. This behavior is part of JavaScript's memory management system and, while not the direct goal of emptying an array, is a consequence of manipulating its `length`.
Efficient Array Emptiness Checks in JavaScript 5 Best Practices for 2024 - Custom isEmpty function implementation
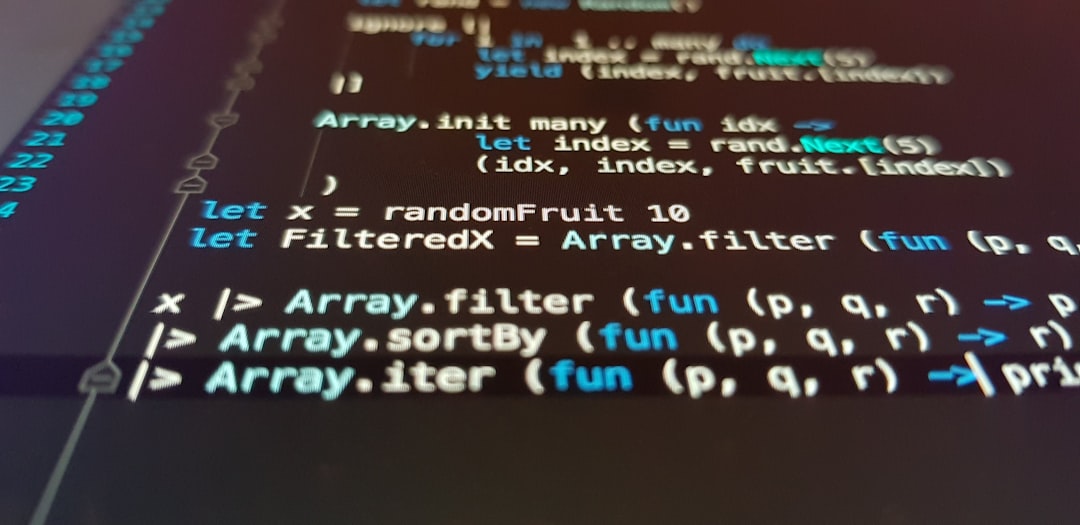
Crafting a custom `isEmpty` function provides a structured and reusable method for verifying if a JavaScript array is empty. The function should begin by employing `Array.isArray()` to confirm that the input is actually an array, thereby mitigating issues arising from non-array inputs like `null` or `undefined`. If the input validates as an array, the function then assesses the `length` property, returning `true` only when its value is zero. Though the implementation is seemingly simple, it's crucial to be mindful of performance implications and potential edge cases, such as the distinction between an empty array and other values that might falsely evaluate to `false`. Building a custom `isEmpty` function fosters improved code clarity and promotes adherence to recommended practices in JavaScript array manipulation. It's an example of how wrapping common tasks within functions improves the robustness and maintainability of code.
1. Crafting a custom `isEmpty` function offers the ability to extend its behavior beyond arrays, potentially defining emptiness checks for other object types. This allows us to introduce array-like behavior to custom data structures, enriching JavaScript's flexibility.
2. Implementing a custom `isEmpty` function provides a more granular control over error handling. It enables developers to tailor responses when encountering unexpected input types, enhancing the overall robustness and resilience of the code.
3. Performance assessments for array emptiness checks can show variability depending on the array's size and the broader context of its use. While the `Array.isArray()` coupled with `length` check boasts O(1) time complexity for smaller arrays, larger datasets or more complex data structures might introduce noticeable delays.
4. A core advantage of creating a custom emptiness check is its adaptability to handle multi-dimensional arrays and nested structures. This significantly increases its versatility, especially when working with deeply structured data.
5. In performance-sensitive situations, utilizing a simple ternary operator within the custom function might offer a marginal performance boost. It avoids function call overhead while maintaining clear and concise logic, a fine-grained optimization worth considering.
6. Although `Array.isArray` and `length` provide efficient solutions, over-complicated emptiness checks can lead to implicit type coercion in JavaScript. This potentially unexpected behavior can lead to inaccurate boolean results, highlighting the need for careful function design.
7. Different web browsers might exhibit unique behavior when handling certain edge cases in `isEmpty` implementations. This underscores the need for rigorous testing across a variety of environments to ensure the function behaves reliably and consistently.
8. A frequent oversight is underestimating the significance of the JavaScript prototype chain when implementing custom emptiness checks. Neglecting to account for the array prototype correctly might lead to misleading outcomes, causing subtle errors.
9. Effectively managing array-like data structures is a crucial aspect of performance optimization. A custom `isEmpty` function can improve performance across different JavaScript frameworks by ensuring that standard array manipulations are consistently optimized, offering a more tailored approach than relying solely on built-in functions.
10. In intricate application scenarios, a robust custom `isEmpty` function can evolve beyond simple emptiness checks. It can be designed to monitor other data states like "null", "undefined", or even implement security checks, adding a deeper layer of data integrity and management to the code.
Efficient Array Emptiness Checks in JavaScript 5 Best Practices for 2024 - Array prototype every method utilization
The `Array.prototype.every` method offers a concise way to determine if every element within an array passes a specific test defined by a provided function. It's particularly useful because it returns `true` for empty arrays, indicating the absence of elements that fail the test. This characteristic can be beneficial in situations where you need to ensure no elements violate a certain condition. However, it's important to remember that extending `Array.prototype` with custom methods can lead to unforeseen issues, including potential performance degradations or compatibility problems with future JavaScript releases. While `every` can be a helpful tool for simplifying array validation, it shouldn't be considered a universal solution. Depending solely on `every` for array-related tasks, especially in performance-critical applications, could result in less-than-optimal outcomes. Therefore, when using `every`, it's essential to be mindful of the broader context and combine it with other recommended practices for array handling to ensure efficient and reliable code. Even though it promotes code clarity and intent, using it thoughtfully alongside other methods and techniques is crucial for achieving optimal results.
Here's a look at some intriguing aspects surrounding the use of JavaScript's `Array.prototype` methods, particularly within the context of array emptiness checks:
1. **Chaining Methods Together**: Combining multiple array methods through chaining offers a neat and efficient way to write code. You can smoothly string together operations like `filter`, `map`, or `reduce` to process data without needing extra variables, resulting in a more streamlined approach to managing array data.
2. **The Risk of Polluting the Prototype**: While adding custom functions to `Array.prototype` can enhance functionality, it introduces the potential for conflicts. This "prototype pollution" can create issues if other libraries or standard JavaScript features rely on native methods. It's crucial to be mindful of compatibility across different environments to avoid unpredictable results.
3. **Performance Can Vary Widely**: The effectiveness of `Array.prototype` methods can change drastically depending on how they're implemented and the array's size. For instance, using a method like `forEach` will take a longer time as the size of your array grows (O(n) time complexity), which can be a significant performance bottleneck for large datasets. It highlights the importance of carefully considering performance when choosing these methods.
4. **Dealing with Sparse Arrays**: When working with arrays that contain `undefined` values, the behavior of `Array.prototype` methods can be interesting. Methods like `reduce` and `map` tend to skip these gaps, potentially leading to unexpected results if not explicitly addressed. So, understanding how these sparse structures affect method execution is essential.
5. **Immutable Code**: Using methods like `slice` or the spread operator can help keep your code free of unexpected side effects by making sure you don't accidentally modify the original array. This can be incredibly useful when dealing with asynchronous environments where state changes might cause unforeseen bugs.
6. **Native or Custom?**: While JavaScript engines are optimized for native methods, custom implementations often introduce inefficiencies. Before creating a custom method that's similar to an existing native function, it's worth thinking about whether it's necessary. Often, using the native methods will offer a more efficient and stable solution.
7. **Compatibility Across Diverse JavaScript Environments**: Not all `Array.prototype` methods are available in every JavaScript environment. Different browsers and platforms might not support the same methods. This is something to keep in mind, especially if you're writing code that needs to run in a variety of contexts.
8. **Error Handling in Functional Methods**: Many of the methods in `Array.prototype` have edge cases that require careful consideration. For instance, calling `reduce` on an empty array without providing an initial value can lead to errors. Having a solid understanding of these edge cases will help you create robust code that handles unusual situations gracefully.
9. **How `Array.prototype` Impacts Memory Management**: The way you use `Array.prototype` methods can have a significant impact on how JavaScript manages memory. For instance, using methods that create many new arrays (like `filter` in a loop) can increase memory usage, potentially causing performance issues. It's helpful to keep an eye on these patterns, especially when developing performance-critical applications.
10. **Customizing Native Methods Through Overriding**: Overriding existing `Array.prototype` methods can be used to add functionality like custom logging or validation. However, this should be done cautiously as it could impact the expected behavior of arrays, making future code maintenance a more challenging endeavor.
Efficient Array Emptiness Checks in JavaScript 5 Best Practices for 2024 - Direct length property check for known arrays
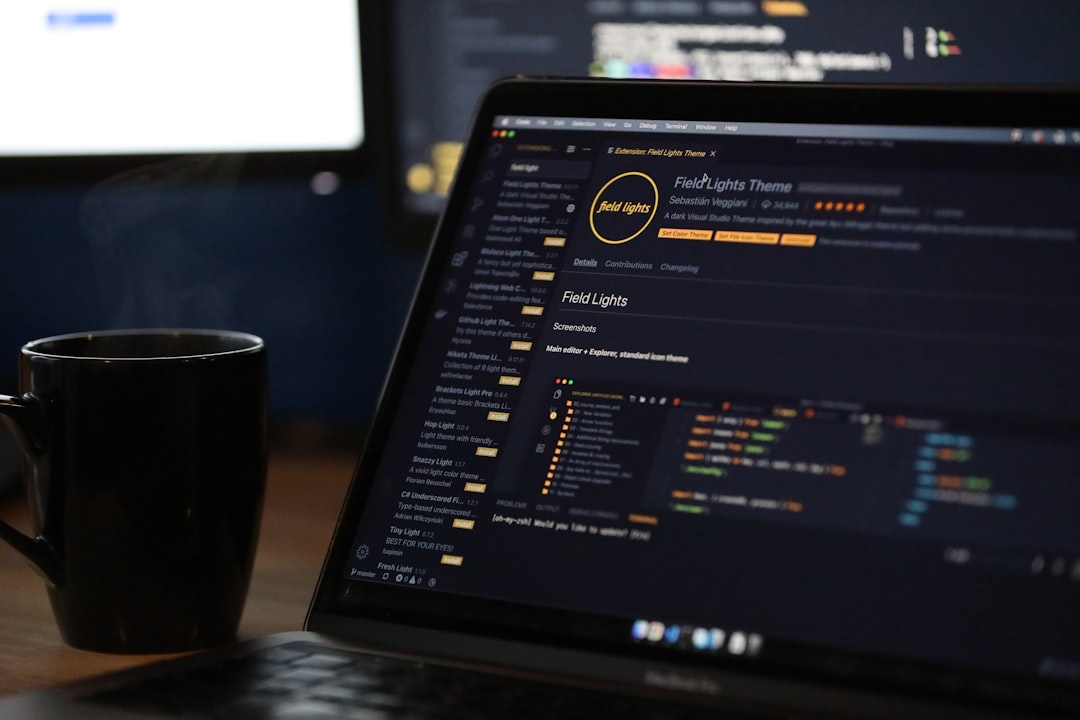
When dealing with arrays in JavaScript, directly checking the `length` property is a highly effective and efficient way to determine if it's empty. This method, relying on a property that JavaScript engines are optimized to access quickly, provides a streamlined approach to evaluating an array's emptiness. By simply comparing the `length` to zero, developers can write concise code that is easy to understand and maintain. It's particularly beneficial when paired with `Array.isArray()` for a comprehensive check. While other techniques are available, they can be more intricate and potentially impact performance negatively. Therefore, employing the direct `length` property check remains the recommended approach for achieving both efficiency and clarity in JavaScript code, particularly as we move towards the ever-evolving landscape of 2024 and beyond. It's a simple, efficient method that, despite its apparent simplicity, offers a powerful tool for maintaining clean and reliable JavaScript applications.
Here are ten interesting observations about directly checking the `length` property of known arrays in JavaScript, which might spark curiosity in developers seeking efficient coding practices:
1. **Balancing Type Safety and Speed:** Combining `Array.isArray()` with a length check offers both type safety and performance. Checking the `length` property happens in a fixed amount of time (O(1)), making it a very fast way to see if an array is empty, particularly important in code that needs to run quickly.
2. **Preventing Unexpected Errors:** Using `Array.isArray()` along with the length check helps stop runtime errors before they happen. If, by mistake, a developer uses something that isn't an array (like a number or a string), the type check will stop it from trying to access the `length` property, which can cause issues if it doesn't exist.
3. **The Changeable Nature of Length:** The `length` property isn't just a mirror of how many elements are in the array; it can be changed. Setting an array's `length` to a lower value can essentially chop it off, potentially even losing some data. This is something to be aware of when working with arrays in JavaScript.
4. **Length Changes and How JavaScript Handles Memory:** Setting an array's `length` to zero doesn't just make it empty; it can also trigger JavaScript's memory management system to clean up elements that aren't used elsewhere in the code. This means your actions have a subtle impact on how JavaScript uses memory.
5. **Performance Impact in Loops:** While checking for emptiness is pretty straightforward, doing it over and over again inside a loop can slow things down. If you repeatedly check if something is an array using `Array.isArray()`, it can add overhead, particularly when dealing with lots of data or frequent checks, which should be optimized.
6. **Balancing Simplicity and Handling Complex Cases:** While the combination of `Array.isArray()` and a length check is simple, more complicated functions might be needed for specific situations. This could be for deeply nested structures or non-standard data types. It shows a trade-off between how easy something is to understand and how well it handles all possible scenarios.
7. **The Pitfalls of Implicit Type Conversions:** Only relying on whether something is "truthy" or "falsy" to evaluate an array's emptiness can lead to errors, since JavaScript's type conversion system can create unexpected results. An empty array (`[]`) is considered falsy, but for clarity, functions that check array emptiness should use direct comparisons.
8. **Maintaining Consistency Across Different JavaScript Environments:** Since `Array.isArray()` was introduced in ECMAScript 5, its implementation has gotten better in different JavaScript environments. It's important to be aware of where your code will run, as older environments might not support this function, and alternative checks might be needed.
9. **Understanding the Prototype Chain's Role:** Modifications or extensions to the prototype chain can affect how checking an array's emptiness works. Though rare, if an object is derived from `Array`, it might have custom behavior that changes how length is seen. This could lead to incorrect results if not managed carefully.
10. **Building for the Future:** Choosing `Array.isArray()` and checking the `length` property ensures your code is ready for any changes to how JavaScript handles arrays. This best practice is part of a broader effort to create stable and predictable code across language updates, ensuring reliability in different scenarios.
Efficient Array Emptiness Checks in JavaScript 5 Best Practices for 2024 - Modern JavaScript syntax for array operations
Modern JavaScript syntax has brought about significant improvements in how we interact with arrays. `Array.isArray()` offers a robust way to confirm whether a given variable is indeed an array, significantly reducing the chances of errors that can occur when assuming a variable's type. JavaScript's dynamic array handling allows for arrays to adjust in size automatically, adapting to changes in the data they hold. Higher-order functions like `map`, `filter`, and `reduce` introduce more functional and concise ways to process arrays, often replacing more verbose looping structures. The adoption of `let`, `const`, and arrow functions contributes to a cleaner code style, making it easier to read, understand, and maintain. These features, in combination, represent a more efficient and expressive approach to array operations, supporting the development of better JavaScript code. While there might be situations where these modern features are not the most suitable, their integration into the language has undoubtedly improved the way we handle and manipulate arrays.
Here are ten interesting points about the modern JavaScript syntax used for array operations, which can help you write better code and understand how things work:
1. The spread syntax (`...`) is handy not only for combining arrays but also for making copies of them. This creates a shallow copy, which is useful for preventing accidental changes to the original array. It's become a popular approach in modern JS development.
2. The rest parameter syntax (`...args`) in function definitions is great for dealing with a variable number of arguments. It automatically gathers all the extra arguments into a single array, which removes the need for complex argument processing inside your functions.
3. `Array.prototype.flat()` (introduced in ES2019) makes it much easier to deal with arrays within arrays. It can flatten the array to a certain depth, saving you from having to write complicated loops or recursive functions.
4. The `includes()` method offers a simpler way to check if an array contains a specific value. It's more direct and readable compared to older techniques like using `indexOf()`.
5. Destructuring arrays allows you to quickly pull specific values out of an array and assign them to variables. This can make your code shorter and easier to maintain compared to manually accessing elements by index.
6. `Array.prototype.findIndex()` is useful for searching an array and finding the first element that satisfies a given condition. It returns the index of the found element, which can be more efficient than looping through the array manually.
7. When using `Array.prototype.map()`, the result is a new array without changing the original one. This is important for preventing unintended changes to the original array, especially in cases where you're managing state.
8. Chaining multiple array methods like `filter()`, `map()`, and `reduce()` can create compact and efficient data pipelines. While it can make the code very readable, it's important to keep an eye on performance, especially with larger arrays.
9. The `entries()` method generates an iterator that gives you both the index and the value of each element in an array. This can be beneficial when you need to work with both pieces of information during iteration.
10. Some developers use currying with array methods to make their code more reusable and flexible. This functional programming technique helps them break down complex tasks into smaller, independent parts.
Learning how to use these modern JavaScript syntax features can help you become more productive and write efficient, maintainable code. It's particularly important in 2024 and beyond, as JavaScript continues to evolve.
More Posts from :