Mastering Java Fundamentals A Step-by-Step Guide for Beginners in 2024
Mastering Java Fundamentals A Step-by-Step Guide for Beginners in 2024 - Understanding Java's Object-Oriented Nature
Java's core strength lies in its object-oriented design. This means it revolves around the ideas of classes and objects, which are fundamental to how Java programs work. Understanding object-oriented programming (OOP) principles like inheritance, encapsulation, abstraction, and polymorphism is crucial for mastering Java. These principles aren't just theoretical; they become truly clear through practical experience. For example, creating your own class, like a Motor Bike class, helps solidify the concepts in a tangible way. Beginners must focus on developing a strong foundation in the basics of Java, gradually progressing towards understanding the intricacies of OOP. Developing a firm grasp of the syntax and standard conventions will smooth the transition to more challenging OOP topics and demonstrate why Java focuses on building reusable and structured code.
Java's core strength lies in its object-oriented design, where the world is modeled as a collection of interacting entities called objects. Each object is an instance of a class, a blueprint that defines the object's attributes (data) and behaviors (methods). Understanding how these classes and objects interact is paramount to mastering Java.
A key aspect of Java's object-oriented nature is encapsulation. This concept essentially guards the internal data within a class, restricting access except through designated methods. While this promotes data integrity and control, it can introduce complexities as it necessitates careful planning when deciding what should be public and what should be hidden.
Inheritance, a powerful yet potentially complex feature, allows for the creation of new classes (subclasses) based on existing ones (superclasses). While this can greatly enhance reusability and reduce redundant code, the resulting class hierarchies can become intricate and, if not managed thoughtfully, lead to confusion.
Interfaces in Java provide a flexible mechanism for specifying behaviors without dictating implementation details. This promotes loose coupling between components, enabling developers to build robust and adaptable systems. However, the need for meticulous adherence to interface contracts can be demanding.
Abstraction is central to simplifying the complexities of real-world systems. By exposing only the essential details of a class or method and concealing intricate implementation, it helps streamline design and enhance comprehension. But relying on abstraction can introduce its own challenges if abstractions become too generalized or poorly defined, leading to a loss of specific functionality.
Polymorphism, the ability for the same method call to perform differently based on the object type, provides dynamic behavior in Java programs. This versatility facilitates elegant interactions and avoids duplicated code for various object types. However, this can make the flow of execution less transparent and potentially more difficult to debug.
Java introduces the concept of immutability with the `final` keyword, offering control over which aspects of classes, methods, or variables can be altered. While this is crucial for building dependable systems, its use requires careful consideration as too many `final` elements can inhibit flexibility.
Java deliberately avoids the complexities of multiple inheritance by preventing direct inheritance from multiple classes. While this decision avoids potential conflicts, it necessitates exploring alternative strategies to achieve desired behaviors via interface implementation.
The Java Virtual Machine (JVM) is a cornerstone of Java's portability and platform independence. It acts as an intermediary, translating Java code into machine-specific instructions, allowing the same program to run on diverse systems. However, this layer of indirection might slightly impact performance in certain situations, making understanding JVM behavior crucial for optimizing code.
The `this` keyword offers a powerful yet subtle way to manage variable naming within a class. It helps disambiguate between instance variables and locally declared variables or method parameters. However, this feature can also complicate code reading for beginners if not understood thoroughly.
Finally, Java's automated garbage collection is a convenience, automatically managing memory allocation and deallocation. However, it can introduce performance variability during runtime, especially in memory-intensive applications. Developers need to strike a balance between the ease of use and potential runtime behavior variations.
Mastering Java Fundamentals A Step-by-Step Guide for Beginners in 2024 - Setting Up Your Java Development Environment
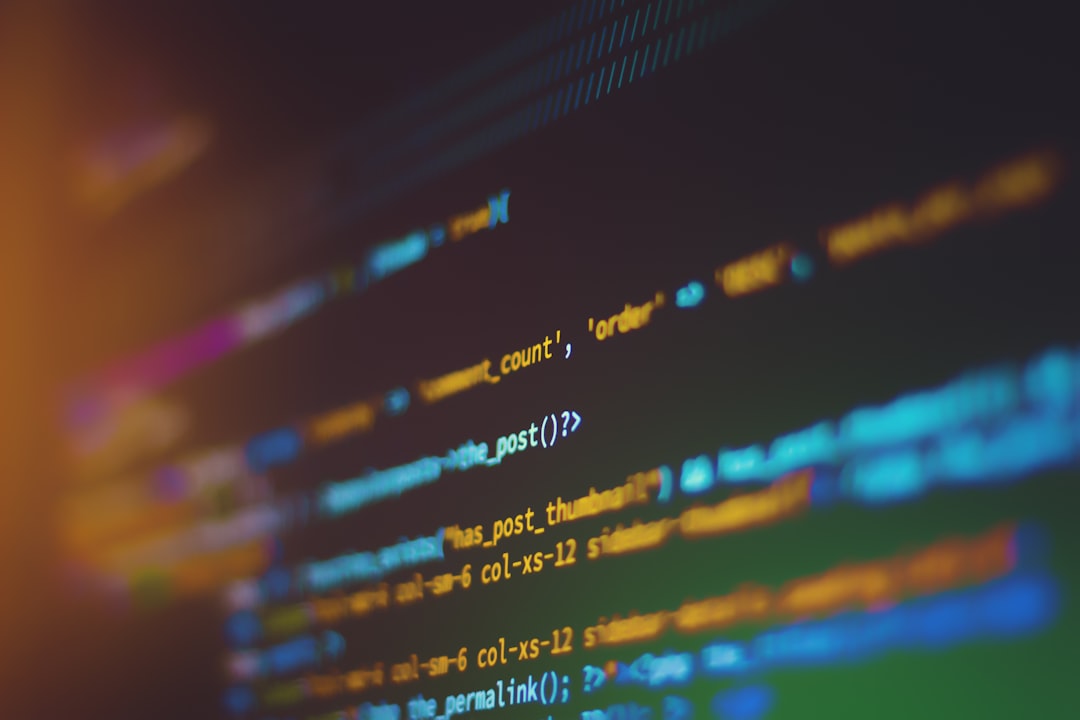
Before you can start writing your first line of Java code, you'll need to set up your development environment. This involves installing the Java Development Kit (JDK), a collection of tools that allows you to write, compile, and run Java programs. Once the JDK is installed, you'll need to adjust your system's environment variables. Specifically, you'll need to point the `JAVA_HOME` variable to your JDK's installation directory and add the JDK's `bin` folder to the `PATH` variable. This ensures your operating system can find and execute Java commands.
To get a feel for the basic structure of Java code, you'll often see beginners start with a simple "Hello World" program. It's a very basic example but helps you grasp the core syntax and structure of a Java program. While getting started might appear to be a few annoying steps, taking the time to properly set up your environment will ultimately pay off, making your journey through the fundamentals of Java much smoother and faster, increasing your productivity along the way.
To effectively embark on your Java coding journey, you'll need to set up a suitable development environment. This involves understanding some key factors that can influence your overall experience. For instance, the system you're using – ideally a 64-bit OS for better performance – will play a part in how smoothly Java applications run. The amount of memory available can be a limiting factor for complex applications.
Moreover, you'll need to carefully choose your JDK version as not all Java tools and libraries work seamlessly across all versions. Picking an outdated or incompatible JDK can lead to confusing behaviors or limitations in what you can achieve.
You'll likely use an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse. These tools offer distinct features, with some compiling code in the background and providing immediate feedback, while others require explicit recompilation, affecting the pace of your coding. While these environments are popular for beginners, a more thorough understanding of how Java works can be gained through the command line interface. This hands-on approach forces you to grasp the intricate details of compilation and execution without relying solely on an automated process.
Setting up your environment involves adjusting certain system settings like `JAVA_HOME` and `PATH` environment variables. These are vital to make sure your command line tools can locate the necessary components of Java, avoiding frustrating errors when you start running your programs.
Since Java code runs on the JVM, a key benefit is its platform independence, however, challenges can arise due to discrepancies in how different operating systems manage files and permissions. Being aware of this can help prevent difficulties.
There's a distinction between the Java Development Kit (JDK) – which contains the tools for building your Java applications – and the Java Runtime Environment (JRE) – which only provides the bare minimum needed to execute them. As a developer, you'll need the JDK for debugging and compilation while end-users only need the JRE to run the applications.
When building larger applications, tools like Maven and Gradle are often employed. These build tools handle dependencies and project structure, simplifying the development process. However, they introduce their own learning curve that can be a challenge for novices.
Furthermore, Java's memory management uses different parts of memory like the heap and stack. Grasping how Java allocates memory in these spaces can help fine-tune application performance and address potential memory leaks.
Finally, to streamline the development process and ensure quality, it's advisable to become familiar with Java's profiling and debugging tools. These are found in various IDEs and can provide insight into performance issues or bugs within the code, shortening your development time. Understanding these tools can help you develop a more robust and efficient application in the long run.
Mastering Java Fundamentals A Step-by-Step Guide for Beginners in 2024 - Writing Your First Java Program
Writing your first Java program is where your journey into the world of Java programming truly begins. The classic "Hello World" program, while incredibly basic, serves as a fundamental stepping stone, helping you grasp the core language structure and basic syntax. You'll discover how a Java program is organized and structured at a rudimentary level. Before you can write that first line of code, setting up your Java Development Environment (JDK) is a necessary preliminary step. This entails installing the JDK and ensuring your system's environment variables, specifically `JAVA_HOME` and `PATH`, are configured correctly. This might seem tedious initially, but it's essential for ensuring that the Java compiler and other tools can be readily found by your system, allowing your programs to execute without unexpected problems.
Moving beyond the initial setup, you'll need to become familiar with data types—how Java represents numbers, text, and other types of information—and how Java handles code execution based on conditions using control flow structures. Mastering these areas forms a solid base for the future. Like any skill, proficiency in Java comes from repeated practice. Engaging daily with concepts like variables, operators, and the flow of instructions in your code will solidify your grasp of the fundamental concepts, paving the way for exploring more advanced Java features as you continue your journey.
### Surprising Facts About Writing Your First Java Program
Java, despite its origins in the mid-1990s, remains a popular programming language. This is due in part to the size and activity of its user communities and the vast collection of readily available libraries, making it appealing to new programmers. Java's strength is its "write once, run anywhere" capability. This impressive feature stems from the way it uses Bytecode, which the Java Virtual Machine (JVM) then interprets for execution. Because of this design, the same Java program can operate on a range of operating systems without any modifications needed.
While Java's syntax may appear relatively easy for those new to programming, the underlying machinery such as garbage collection and just-in-time compilation adds significant layers of complexity that often yield impressive performance. We can often see new users writing a "Hello World" program. This, while simple, introduces the key elements of Java's syntax. It is a practical way to immediately work with core components of Java like classes and methods.
The execution of Java code is a hybrid of compiled and interpreted programming. The Java compiler converts code into Bytecode, and then the JVM handles the interpretation. This unique approach provides a balance between execution speed and the flexibility to run across many different kinds of computing hardware.
Java's automatic memory management, known as garbage collection, does simplify the programmer's experience. However, it also introduces some interesting quirks into how performance occurs over time. Java garbage collection can automatically handle memory leaks, a problem that lower-level languages frequently struggle with.
The Java language has evolved quite a bit since its early versions, continuously being updated and improved. Updates have brought features that increase performance and usability such as lambda expressions introduced in Java 8. These changes highlight the ongoing commitment to adapting Java to modern development practices.
One of Java's primary strengths is its expansive standard libraries. These libraries are pre-built collections of tools covering everything from networking to handling data. This is extremely helpful to new programmers who can benefit by leveraging ready-made tools rather than needing to build the same functionalities from scratch.
Java's object-oriented approach provides many advantages but has the possibility to lead to overly elaborate designs if not managed correctly. This is an important lesson for new users who often learn early on that simpler solutions usually lead to code that is easier to maintain.
The initial phase of learning Java can be more demanding than some other languages due to its strong emphasis on typing and its object-oriented nature. However, this initial challenge is a worthwhile opportunity to develop valuable skills such as critical thinking and problem-solving – both essential aspects for becoming a proficient programmer.
Mastering Java Fundamentals A Step-by-Step Guide for Beginners in 2024 - Exploring Java Data Types and Variables
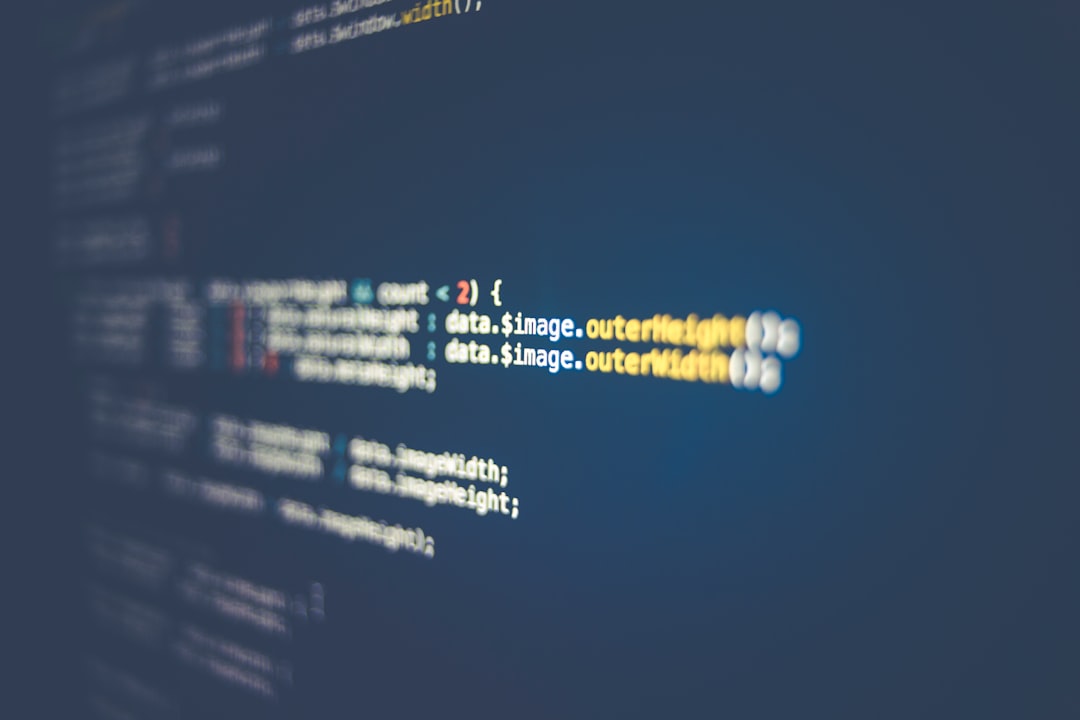
In the world of Java, understanding data types and variables is fundamental. Data types define how Java stores different kinds of information, like numbers or text. The `int` data type, for instance, is designed to hold whole numbers within a specific range, making it suitable for counting or representing quantities. Variables act as containers for storing this data. They provide a way to label and manipulate information throughout your program, allowing you to build more complex applications.
Gaining a solid understanding of how data types and variables interact is vital. You'll want to become fluent in the different ways Java stores information. While the concept might seem straightforward initially, becoming comfortable with variable declaration and manipulation is essential for building more complex applications later on. Hands-on exercises can significantly help beginners internalize these concepts, preparing them for the more advanced elements of Java programming.
As you delve deeper into Java, you'll discover that the ability to effectively work with data types and variables significantly influences your code's clarity, efficiency, and overall robustness. It forms the basis for more advanced concepts and techniques you'll encounter further down the road, making a strong foundation in this area a worthwhile investment for any aspiring Java developer.
Delving into Java's data types and variables is like exploring the fundamental building blocks of the language. Java utilizes eight primitive data types, such as `int` (a 32-bit integer) and `double` (a 64-bit floating-point number), that are fixed in size and consistent across platforms. While this promotes consistency, it can sometimes limit flexibility when dealing with a wider range of numeric values.
Java also features automatic boxing and unboxing, which automatically convert between primitive types and their corresponding wrapper classes like `Integer` and `Double`. This seemingly convenient feature can sometimes introduce an overhead penalty, particularly in performance-sensitive code where frequent conversions occur.
The scope of a variable, the region within a program where it can be accessed, is a significant concept in Java. A variable's scope can be local to a method or belong to a specific object instance, showcasing the object-oriented nature of the language. Understanding scope is vital for effective memory management and preventing unintended variable access.
Java's `String` class is immutable, meaning once created, its content cannot be altered. While this promotes data integrity, it can lead to unexpected performance impacts as new `String` objects are created for seemingly minor changes. There is a trade-off between the benefit of stability and the added overhead of constant object creation.
Enumerated types, or enums, were introduced to define a set of related constants. Interestingly, these enums can include methods and attributes, essentially behaving as classes. They provide a structured mechanism to handle a pre-defined collection of values, enhancing clarity and readability in particular areas of code.
Java has a rule about initializing variables. If you do not explicitly give a variable an initial value, it will receive a default value based on the data type, like 0 for integers. This behavior, while useful for memory management, can easily lead to unexpected bugs if a developer mistakenly assumes a particular default value or if it is not obvious.
The `var` keyword introduced in Java 10 allows type inference, allowing a programmer to skip explicitly declaring the type of a variable. While this can streamline code, overusing it can cause confusion when attempting to understand the code's functionality, as it can be unclear which inferred type was applied.
Binary literals, introduced in Java 7, let programmers represent integers in their binary form, making it easier to understand the code in situations where binary values are crucial, such as in low-level programming. This feature simplifies understanding code that involves bit manipulation.
The `final` keyword in Java makes variables constants. Their values can only be assigned once, promoting code clarity. However, using `final` too liberally can lead to inflexibility, as code often requires the ability to update the values of some variables. It is often a balancing act between rigidity and adaptation.
Unlike certain programming languages, Java doesn't permit operator overloading. In essence, this means you can't redefine the behavior of operators for custom data types. While it simplifies Java, it reduces the potential for developers to create highly expressive syntax, a tool that might be expected in some other programming contexts.
Mastering Java Fundamentals A Step-by-Step Guide for Beginners in 2024 - Mastering Control Flow Statements in Java
In your journey to become proficient in Java, mastering control flow statements is fundamental. These statements are the mechanisms that enable your Java programs to make decisions and control the order in which instructions are executed. Statements like `if`, `if-else`, and `switch` provide the means to direct execution based on various conditions, essentially giving your code a branching capability. This is a powerful feature that's core to creating dynamic and responsive applications.
Moreover, loops such as `for`, `while`, and `do-while` equip your programs with the ability to repeat certain blocks of code, leading to more efficient code and fewer repetitive lines. This ability to iterate through sections of code is critical to automating tasks and making your programs more versatile.
Understanding how to use these control flow constructs is essential for both beginning and advanced programmers. It unlocks the ability to write sophisticated code that handles various situations. Whether you're just starting out or have some Java experience, tackling the control flow statements and seeing them in action through practical examples is the best way to solidify your learning. Applying these concepts to coding challenges is a fantastic way to develop real-world problem-solving skills and to become more confident and competent in developing Java applications.
Control flow statements in Java are fundamental for directing the execution path of a program, essentially deciding which parts of the code run based on certain conditions. They are the building blocks that allow Java to be more than just a sequence of commands executed top to bottom.
Common control flow mechanisms include the `if`, `if-else`, and `switch` statements, which handle decision-making in the code. These statements offer ways to execute different parts of a program depending on whether a condition is met. Loops, including `for`, `while`, and `do-while`, let code blocks be repeatedly executed until a condition becomes false, making it ideal for repeating actions or processing collections of data.
Control flow is crucial for shaping how a Java program behaves, influencing its adaptability and allowing it to respond to changes in the environment or user input. It introduces branching and iterative capabilities into the program flow, shifting it away from a linear path. Both newer and experienced developers must fully understand how these control structures affect the execution order.
Java seamlessly integrates control flow using conditional statements and looping constructs. Understanding these foundational elements is key for efficiently implementing intricate logic in Java programs. In teaching Java to new programmers, understanding control flow is crucial as a core component of the language. It's usually a significant part of many Java learning resources such as tutorials and courses.
Control flow fundamentally alters the default top-to-bottom sequence of execution. It enables the program to navigate to different blocks of code based on results or repeat a section of code many times. Many introductory resources use practical examples and code exercises to illustrate how to utilize these constructs, fostering a deeper comprehension of their applications in real-world programming scenarios.
While conceptually straightforward, control flow statements can be unexpectedly tricky. This can be due to the way they interact with other components of Java or because the language itself has evolved to offer more and more sophisticated constructs over time. When used incorrectly, the result can be confusing or difficult to debug. For example, relying on exceptions for program logic can hurt performance and readability. It is vital to understand the trade-offs inherent in these powerful tools. While simple in concept, they underpin the ability to create powerful Java applications. It's a continuous journey for programmers to keep mastering these building blocks.
Mastering Java Fundamentals A Step-by-Step Guide for Beginners in 2024 - Implementing Object-Oriented Programming Concepts
Implementing the core principles of object-oriented programming (OOP) within Java is fundamental for building software that's well-structured, easily expanded, and simple to maintain. Key ideas like encapsulation, inheritance, and polymorphism are powerful tools that help developers model real-world scenarios and interactions using objects. Encapsulation protects the internal workings of an object, ensuring data integrity and promoting a clear interface for interacting with it. Inheritance allows you to build on existing code, creating new classes based on previously defined ones, boosting code reusability. Polymorphism, on the other hand, introduces the ability for the same method to operate differently depending on the specific object it's applied to, enhancing the adaptability and versatility of your code. Understanding these principles not only leads to better software design but also provides a valuable way for beginners to understand how complex systems can be modeled and broken down into manageable components. However, keep in mind that these OOP concepts can also add significant complexity to your projects. Careful consideration and meticulous design are essential to avoid building software structures that are needlessly convoluted and difficult to comprehend.
Java's object-oriented approach, while powerful, presents its own set of intricacies. The core OOP principles—encapsulation, inheritance, abstraction, and polymorphism—though initially intuitive, can lead to unforeseen consequences if not carefully considered.
Encapsulation, while intending to protect data integrity, can make debugging more challenging by shielding internal object details. This "information hiding" can create obstacles when developers need access to critical data for troubleshooting. It can sometimes seem as though encapsulation prioritizes hiding information over facilitating clear debugging, which can lead to complexities.
Inheritance, a valuable tool for code reuse, can easily lead to complex class hierarchies that become challenging to understand and maintain. Instead of deep inheritance structures, preferring composition—combining objects—could provide clearer relationships between classes, making code easier to comprehend. This choice can lead to a better balance between reusability and maintainability.
Java's interfaces, while promoting flexibility by defining behavior without implementations, can create an over-emphasis on behavioral design if not used carefully. It's important to consider that interfaces lack a mechanism for defining state, which could cause over-design or excessive abstraction if not used judiciously. Striking a balance between loose coupling and the inherent limitations of this approach can be challenging.
Abstraction simplifies complex systems by revealing only essential details. However, this simplification might mask performance-related issues that can be difficult to detect when relying on highly abstract layers. When performance is a major requirement, understanding the underlying implementations can become vital to optimize the software's behavior. This relationship between the benefits of abstraction and the constraints it can create is a notable tradeoff that Java developers face.
Polymorphism offers flexibility in how methods interact with different objects. However, this dynamic behavior has a runtime overhead associated with method resolution. It's beneficial to be mindful of potential performance hits, particularly in sections of code where execution speed is paramount. It is often a fine line between the benefits of versatile code and the efficiency needed to produce responsive systems.
Immutability, a cornerstone of Java's object model, as seen with the `String` class, ensures that objects cannot be modified after their creation. This feature makes multi-threaded applications easier to develop by avoiding shared state complexities. But this strict design constraint leads to substantial object creation, which can increase memory usage over time. Developers need to weigh this gain in reliability against the additional costs it creates.
The `final` keyword, when applied to classes or methods, prevents extension or overriding. This provides a way to enforce stability, but its over-use can compromise future flexibility. There is often a delicate balance between limiting flexibility to maximize stability and retaining the ability to adapt code as the needs of an application change.
Java's fundamental structure necessitates that all classes inherit from the `Object` class, granting all objects a standard set of methods like `equals()` and `hashCode()`. While this design promotes uniformity, it can lead to potential confusion when attempting to override these methods in subclasses. This unified approach can sometimes create confusion, especially for developers still coming to grips with how object hierarchies are managed in Java.
The convenience of automatic garbage collection can lead to memory leaks if developers don't manage references between objects thoughtfully. For example, complex object relationships may lead to scenarios where the garbage collector does not reclaim memory, especially in situations where references are incorrectly held or are more complex. While this removes the burden of manual memory management, it does require a deeper understanding of how Java memory management works.
These complexities are vital to grasp, reminding us that the seemingly simple elegance of Java's object-oriented model necessitates diligent development practices. It's this understanding that elevates Java developers from merely writing code to creating robust, efficient, and scalable applications. It's a continuous journey of refinement where the strengths and potential pitfalls of the language must always be kept in mind.
More Posts from :