Mastering JavaScript's RegExp Replace A Deep Dive into Efficient String Manipulation
Mastering JavaScript's RegExp Replace A Deep Dive into Efficient String Manipulation - Understanding the basics of JavaScript's RegExp object
Before we dive into the intricacies of `replace` within JavaScript's RegExp, it's crucial to grasp the fundamental nature of the RegExp object itself. JavaScript offers two approaches for constructing regular expressions: literal notation, employing slashes to enclose the pattern (e.g., `/pattern/`), and the `RegExp` constructor, utilizing a function-like syntax (e.g., `new RegExp('pattern')`). Each approach has its own niche, and understanding the difference can enhance the readability and maintainability of your code.
At its core, a regular expression is nothing more than a structured sequence of characters defining a specific search pattern. This capability opens doors to a vast array of applications, including data validation, parsing text data structures, and manipulating strings in various sophisticated ways. The RegExp object comes equipped with several useful methods, including `exec` and `test` for pattern matching and a host of string methods like `match`, `replace`, `search`, and `split` that provide a powerful toolkit for text manipulation.
However, mastering RegExp is not just about familiarity with the methods. Understanding the underlying syntax is just as important. This includes delving into elements like character classes, quantifiers, assertions, and flags—all of which enable the creation of increasingly sophisticated and flexible patterns. Mastering the use of optional flags like `i` for case-insensitive searches, `g` for global matches, and `m` for multiline searches further adds to your arsenal of string manipulation techniques. These are subtle but powerful features that unlock efficient string handling in JavaScript. Essentially, proficiency in regular expressions can transform how you work with strings, offering a compelling avenue to boost development efficiency and productivity.
1. JavaScript's regular expression engine offers two approaches for defining patterns: literal notation, like `/pattern/`, and the `RegExp` constructor, `new RegExp('pattern')`. The constructor allows for patterns built from variables, increasing the flexibility of regex usage, particularly in dynamic scenarios.
2. While JavaScript's regex implementation defaults to case-sensitive matching, the `i` flag can be used to make it case-insensitive. This can be helpful, but it's important to be mindful that this may produce unexpected results if not carefully managed during string manipulation operations.
3. It's worth noting that regular expressions can exhibit varying performance depending on their complexity and the length of the input strings. Overly intricate patterns can sometimes lead to "catastrophic backtracking" due to the engine trying to find matches, which significantly slows things down.
4. JavaScript's RegExp objects incorporate several predefined character classes, like `\d` for digits and `\w` for word characters, which simplifies the construction of common character patterns. This can save time and reduce the risk of errors compared to defining them explicitly.
5. Capture groups, represented by `(...)`, provide a way to retrieve text matched by the regex but can also be used in conjunction with quantifiers, adding an element of conditional logic into pattern matching that allows for more refined and intricate scenarios.
6. The `g` flag is essential for finding every instance of a pattern within a string, as the regex engine, by default, halts after finding the first match. This flag can be critical in scenarios where it's important to operate on every matching substring.
7. The "sticky" `y` flag has a specific behavior: it ensures the regex engine only starts a match at the current position within the string, thereby avoiding unintentional matches and ensuring precise alignment to a specific location within the input string.
8. While regex patterns can express complex search criteria efficiently, it can come at a cost: the patterns themselves might become difficult to read and maintain, especially when projects involve collaboration. Writing well-commented regex patterns can help address this.
9. When employing the `replace()` method to replace patterns with new strings, keep in mind it's critical to consider the `g` flag. Without it, only the first instance of the pattern is altered. Therefore, to ensure every match is accounted for, utilizing the global flag is usually necessary.
10. The fact that JavaScript allows you to build and adapt regular expressions dynamically can be useful for testing and debugging. You can dynamically construct and refine your patterns as you're experimenting and iterating, which is a powerful feature for adapting to varying data structures or input types.
Mastering JavaScript's RegExp Replace A Deep Dive into Efficient String Manipulation - Exploring the power of the replace() method in string manipulation
Delving deeper into JavaScript's string manipulation capabilities, we encounter the `replace()` method, a powerful tool for finding and substituting specific patterns within strings. This method operates non-destructively, creating a new string with the replacements applied rather than modifying the original. By default, `replace()` targets only the first instance of the pattern found. However, utilizing a regular expression with the global (`g`) flag allows you to replace all occurrences of the pattern, expanding the scope of its utility.
Beyond basic replacements, the `replace()` method's flexibility extends to incorporating a function as its second argument. This allows for dynamic substitutions, making decisions about the replacement based on the specific match found. This added functionality underscores the versatile nature of `replace()` in various string manipulation tasks.
While `replace()` is undoubtedly a valuable asset, it's crucial to be aware of its limitations. For exceptionally complex string manipulations, you may find that it falls short, necessitating the use of other techniques for achieving the desired results. Understanding these boundaries helps ensure you choose the most appropriate tool for the job.
The `replace()` method offers a dynamic approach to string manipulation through the use of a callback function as its second argument. This allows for customized replacement logic based on the captured substring, expanding the method's versatility. However, it's important to note that if you're using a string as the replacement value and it includes special characters like the dollar sign (`$`), these are interpreted as placeholders for captured groups. This behavior, if not properly considered, can produce unintended results.
Crucially, `replace()` doesn't alter the original string. Instead, it creates a new string incorporating the replacements. This characteristic is aligned with JavaScript's broader approach to strings as immutable data types, ensuring that the original string remains intact for any further operations.
When dealing with regular expressions within the `replace()` method, the sequence of replacements matters. If you have multiple replacements defined in a single call, earlier matches might change how later matches are interpreted because of the evolving structure of the resulting string. This can have subtle but significant consequences if not carefully considered.
We can effectively expand the core replacement functionality by chaining together multiple `replace()` methods. This allows for more complex transformations on a string, but we need to be aware of potential performance bottlenecks caused by the repeated processing overhead of the replacement operations.
One aspect where JavaScript's `replace()` deviates from some other languages is the lack of support for advanced regex features such as lookbehind assertions, at least until the ECMAScript 2018 standard. This limitation can mean needing alternative strategies when tackling more intricate pattern matching scenarios.
Furthermore, while the global flag (`g`) is useful for replacing all instances of a pattern, the engine, by default, will only backtrack if `g` is provided. If you're aiming for a global replacement, it's crucial to ensure the `g` flag is present in the regular expression to prevent accidental misses.
Regular expression matching in JavaScript is case-sensitive by default. When employing `replace()`, this sensitivity can be a deciding factor in the outcomes. For instance, if you use `replace(/pattern/i, 'replacement')`, you're enabling case-insensitive matching, but this also needs to be well-understood in the context of your text to prevent undesired modifications.
As with any string operation, performance can be affected by input size and the complexity of the regular expressions used. For cases with large strings or intricate patterns, it might be helpful to precompile regular expressions to optimize performance by reducing repeated pattern parsing and matching steps.
Finally, `replace()`'s ability to reference captured groups within the replacement string offers a pathway for complex manipulations of the matched portions of the text. This opens possibilities for sophisticated text rearrangement or transformation, enhancing the power of string manipulation within JavaScript.
Mastering JavaScript's RegExp Replace A Deep Dive into Efficient String Manipulation - Advanced pattern matching techniques using RegExp flags
Beyond the fundamental aspects of regular expressions, JavaScript's RegExp flags unlock advanced pattern matching capabilities that greatly enhance string manipulation. Flags like `i` for case-insensitive matching, `g` for global searches, `m` for multiline patterns, and `y` for sticky matches, significantly change how a regular expression behaves. These seemingly small additions to your patterns enable finer control over matching, allowing you to efficiently find all instances, ignore casing, work with multiline text structures, or control the exact starting point of matches within a string.
However, while these flags expand the expressive power of regular expressions, they can make patterns harder to read and understand, especially in larger projects. It's important to be mindful of this potential for complexity when using flags, especially when working with others on a project or debugging intricate patterns. Carefully crafting well-commented regular expressions can mitigate this, but the need for caution and clear understanding is always present. Ultimately, proficiency with RegExp flags provides a crucial set of tools for those looking to refine and optimize string manipulation practices within their JavaScript development.
Regular expression flags in JavaScript aren't just about modifying how patterns are matched, they can fundamentally change the behavior of the regex engine. For example, the `m` flag alters how the beginning (`^`) and end (`$`) anchors work, making them match the start and end of each line instead of the entire string. This is useful when you need to process text line by line.
While the `i` flag makes matching case-insensitive, it's worth noting it can sometimes impact performance. When dealing with a substantial amount of data, this extra logic can unexpectedly slow down string operations. It's a tradeoff you need to consider, especially for performance-sensitive tasks.
The interaction between the `g` and `i` flags is important. If you're dealing with a string where a single pattern might have variations in case, being mindful of how these flags work together will ensure replacements don't introduce unintended errors.
For handling text with Unicode characters, which is increasingly common in global applications, the `u` flag is essential. It ensures that the engine correctly interprets these characters, preserving accuracy in your match and replacement operations.
The `s` flag, or dot-all mode, can be a boon when processing multiline text. By allowing the dot (`.`) to match newline characters, you avoid situations where the engine's default behavior prevents matches across lines. This can streamline tasks involving complex multiline text.
If you're utilizing capture groups in conjunction with the `g` flag, keep in mind that omitting the `g` flag causes `replace()` to only capture the first match. If your logic assumes that every instance is captured and manipulated, forgetting this can lead to unexpected consequences in your string structure manipulations.
While powerful, relying on regex for string manipulation also comes with security concerns. Improperly sanitizing user input before using it to build regex patterns can create a pathway for Regular Expression Denial of Service (ReDoS) attacks. These attacks leverage inefficient regex performance to cause issues, so it's worth being aware of and mitigating.
Backreferences in regex patterns are helpful for complex string manipulation, but overusing them can hinder readability and make future maintenance more challenging. In collaborative environments, this increased complexity can lead to more confusion and errors.
The `y` flag, which only attempts matches at the current position, offers a unique way to pinpoint specific parts of a string. It offers greater control over matches in intricate situations, but its behavior is a bit less intuitive than the more commonly used flags.
Even with their considerable power, regex might not be the most effective solution for every string manipulation task. Simple string operations might be handled with greater clarity and efficiency by standard string methods. Knowing when to apply the right tool for the job can lead to more robust and understandable code.
Mastering JavaScript's RegExp Replace A Deep Dive into Efficient String Manipulation - Leveraging capture groups for complex string transformations
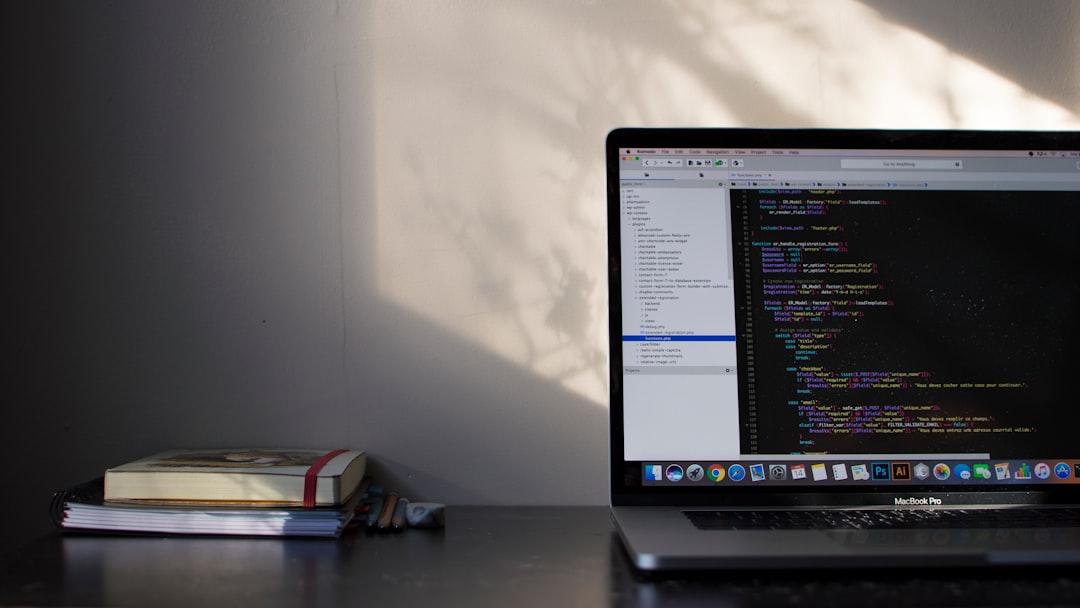
Capture groups, defined using parentheses `(...)` within regular expressions, provide a mechanism for performing intricate string transformations in JavaScript. These groups allow you to isolate portions of a matched pattern and subsequently reference them within the replacement string using constructs like `$1`, `$2`, and so on. This enables you to restructure parts of a string, rearrange elements based on the captured patterns, or format data according to specific conditions.
For instance, you could use capture groups to extract specific parts of a date string and rearrange them into a different format. The power of capture groups extends to the ability to nest them, creating increasingly complex patterns. However, this added complexity can impact the readability and maintainability of your regex, making it crucial to prioritize clarity and consider the performance implications.
In essence, capture groups enhance your string manipulation toolkit, opening doors to a wider array of transformations, but it's important to use them thoughtfully, balancing the power they offer with the potential complications they introduce.
1. Capture groups aren't just for extracting parts of a match; they can also improve performance by avoiding repetitive pattern definitions. Clever use of groups lets you express complex searches concisely, which can make a difference when dealing with large chunks of text.
2. Each capture group gets a number based on its order in the regex, starting from 1. Being able to refer to these numbered groups in the replacement string allows for some really intricate string shuffling. You can dynamically transform text based on the parts that match your pattern.
3. JavaScript's regex engine lets you nest capture groups, which means you can have groups inside other groups. This gives you the ability to finely control how matched parts are handled, but it can make the pattern itself harder to understand and can complicate how you analyze performance.
4. The replacement string you use in the `replace()` method is directly linked to the structure of the capture groups in the regex. If you change the regex, you may have to adjust the replacement logic to keep everything working correctly. This is something to keep in mind when you're maintaining your code to ensure it behaves as expected.
5. You can use non-capturing groups (`(?:...)`) to improve how easy it is to read the regex without affecting what gets replaced. These groups don't store the matched text for later use, but they can help organize complex expressions without having extra group indices to deal with.
6. Using positive lookaheads (available in JavaScript since ECMAScript 2018) lets you check for more complex matching conditions without actually including the lookahead in the replacement. This is helpful for fine-tuning replacements based on context without cluttering up the output string.
7. Capture groups can interact in unexpected ways with different regex flags. For example, using both the global (`g`) and multiline (`m`) flags can change how capture groups work and affect what parts of the string are replaced. It's a detail that needs careful attention when developing regex-based replacements.
8. You can use backreferences in replacements to cross-reference different captured groups and produce very specific outputs. While powerful, this can complicate the regex itself and make debugging and validation trickier. It's essential to find a balance for maintainable and understandable code.
9. The more capture groups you have, the greater the chance of mismatches, particularly if there are overlapping or similar patterns. When patterns get complex, thorough testing and validation are crucial because the consequences of unintended replacements can be severe.
10. Complex regexes with a lot of capture groups can be computationally expensive, especially with large datasets. When you're building transformations, it can be helpful to benchmark different approaches to see which patterns are the most efficient for your specific situation.
Mastering JavaScript's RegExp Replace A Deep Dive into Efficient String Manipulation - Optimizing RegExp performance in large-scale applications
When dealing with large-scale applications, optimizing regular expression (RegExp) performance is paramount for ensuring smooth and responsive user interactions. A common issue that impacts performance is the repeated compilation of regex patterns inside frequently executed functions. Placing those patterns outside those functions avoids the overhead of recompilation, leading to faster execution.
Additionally, understanding how to prevent "catastrophic backtracking" and the advantages of using possessive quantifiers is important when designing complex patterns. Catastrophic backtracking can occur when the regex engine struggles to find a match, drastically slowing down the process. Possessive quantifiers, while not always straightforward to implement, can provide a way to improve the matching speed under certain circumstances.
Further enhancing performance, especially when working with intricate patterns and massive datasets, is choosing engines that utilize the Thompson NFA algorithm. While not always available in all environments, these engines provide a more efficient method for processing regular expressions.
Ultimately, balancing the power and flexibility that regex offers with the importance of maintainable code is key. Overly complex regular expressions can severely impact both performance and readability. Finding the right level of complexity for the task at hand—and striving for clarity in the regex pattern itself—is a crucial aspect of achieving optimal RegExp performance in your application.
1. Regular expressions can be surprisingly demanding on memory, particularly when they involve backreferences or deeply nested capture groups. In large applications, if these complex patterns are used repeatedly, it can lead to significant memory usage and slowdowns. This suggests we should be mindful of the memory footprint of our regexes, especially in resource-sensitive scenarios.
2. JavaScript engines often apply optimizations to simpler regular expressions, so patterns like `/abc/` can often be faster than more complex ones. Understanding these optimizations can inform our design choices, particularly when performance is crucial. This suggests that simpler regexes, when possible, are preferable in performance-critical contexts.
3. While regex is a powerful tool, it's easy for it to become a performance bottleneck if not carefully handled in large applications. Complex patterns, especially ones that trigger extensive backtracking, can really slow things down. This highlights the need for careful regex design to avoid performance problems that can arise in large-scale applications.
4. The `u` flag, which activates Unicode support, adds a layer of intricacy when handling non-ASCII characters. If we're not careful about how these interact with our patterns, it can lead to some unexpected results. This indicates that Unicode support needs careful consideration and testing when dealing with potentially multilingual datasets.
5. Regular expressions can create security risks like ReDoS attacks, where a carefully crafted input can cause the regex engine to consume excessive resources or time. This emphasizes the importance of input sanitization before using it to build regex patterns. This highlights a crucial security consideration: input validation is essential for preventing regex-based security vulnerabilities.
6. The performance effects of regexes can vary a lot based on the complexity of the pattern and the data they're working with. Testing has shown that even with the same pattern, variations in the input can lead to differences in execution time on the order of hundreds of milliseconds. This means profiling and careful performance testing is often necessary when using regex in performance-critical applications.
7. We can often improve the efficiency of regex operations by precompiling the expressions if they are going to be reused frequently. This can be a big performance boost by avoiding the overhead of parsing the pattern every time it's used. This suggests that precompilation is a good optimization strategy for common regex patterns.
8. When combining `replace()` with regex in JavaScript, it's crucial to realize that dynamic string changes caused by the replacement can affect how subsequent regex evaluations happen. This can lead to unforeseen outcomes, emphasizing that we need to be aware of the interplay between string modifications and regex during development. This means we must carefully consider how dynamic string changes due to `replace()` will impact future regex usage.
9. The `s` flag, which makes `.` match newline characters, is helpful for handling multiline strings. However, it also means it's easier to get unintended matches. This can lead to some tricky debugging situations with complex patterns. This means we must be aware that the `s` flag can significantly alter the matching behavior and needs careful consideration in its implementation.
10. Thoroughly testing and validating complex regex patterns is essential, since errors in nested capture groups or flag usage can be difficult to spot. Having a comprehensive testing strategy, especially covering edge cases, can prevent costly issues in production. This emphasizes the critical importance of robust testing, including thorough edge-case validation, to ensure correct regex behavior.
Mastering JavaScript's RegExp Replace A Deep Dive into Efficient String Manipulation - Real-world examples of RegExp replace in web development projects
Within the realm of web development projects, the `replace()` method, powered by regular expressions, becomes a cornerstone for effectively manipulating strings. Its applications are diverse, ranging from refining user input for consistent formatting to safeguarding data through sanitization, or dynamically producing content based on identified patterns in strings.
For instance, a common scenario involves restructuring date formats, like transforming "MM/DD/YYYY" into "YYYY-MM-DD". This task often leverages capture groups to isolate and rearrange the different parts of the date string. Similarly, developers often rely on regex replace to eliminate extraneous characters from input fields, such as superfluous whitespace or enforcing specific data formats. This practice ensures data integrity before any processing takes place, making applications more robust.
Proficiency in applying regex replace not only accelerates application performance but also leads to cleaner and more sustainable code. A deeper understanding of these practices can result in applications that are easier to maintain and more reliable over the long run.
1. In extensive web applications, a common use of `replace()` is tidying up and standardizing user-provided data. For example, removing unnecessary characters from phone numbers or making sure dates are formatted consistently. This makes sure data is reliable throughout different parts of the application, simplifying processing and storage.
2. It's interesting that `replace()` can be part of real-time feature development, like changing parts of code in a live-updating development environment based on user interactions. This allows for faster development loops and immediate feedback.
3. While regex is powerful for string manipulation, using it too much can lead to code that's hard to read and maintain due to excessively complex patterns. Teams find that using basic string methods can often make collaboration and understanding easier among developers.
4. Web developers often use `replace()` to clean output before it's displayed in a browser. This can involve turning HTML characters into safe forms to prevent attacks like Cross-Site Scripting (XSS), showing how regex is useful for both features and security.
5. Interestingly, many text editors use `replace()` with regex to provide advanced search and replace options. This enables users to quickly manipulate large amounts of text, highlighting regex's flexibility outside traditional coding contexts.
6. Developers frequently use `replace()` with capture groups to pull information from log files or comma-separated value (CSV) files. This helps break down structured text into organized data. By selecting parts of the input string, they change messy log data into JSON objects, which makes it easier to analyze.
7. Another clever use is in internationalization, where regex helps to translate content on the fly. By replacing specific phrases or variables with different language equivalents, developers streamline the localization process, improving the user experience for people all over the world.
8. While typically optimized, complex regex structures can occasionally lead to unexpected behavior during replacements, especially when using lookaheads or multiple flags. Thorough testing with various datasets is crucial to guarantee the expected results across a wide range of situations.
9. Many developers leverage `replace()` to generate unique identifiers or slugs from strings, changing spaces and special characters into formats that are appropriate for web addresses. This transforms user content into clean URLs, improving search engine optimization (SEO) and ensuring consistent website navigation.
10. Notably, using `replace()` with a function as a second argument enables complex conditional replacements based on the text that matches the regex. This is useful for situations where you need to apply multiple replacement rules dynamically, enhancing the flexibility of text manipulation.
More Posts from :